General
This is the reference for the SimpleMES application. The goal of this document is to provide in-depth details on most aspects of the application. The Groovydoc (Javadoc) API covers other details of the underlying classes, but this document explains the higher-level features and behavior.
For a high-level treatment of these features, see the User Guide.
Conventions
This document follows certain conventions as shown below:
-
Literal values
-
File/path names
-
Class names
-
Service names
-
Variables, fields or parameters
-
Method names
-
Groovy or java code
The application follows certain naming conventions:
-
Utils
- Miscellaneous utility methods, usually static methods that make your life easier. -
Helper
- A helper class that has a more complex interface. These usually do not use static methods to access the functions.
See also Framework Introduction for more conventions.
Standard Fields
Almost all persistent objects in SimpleMES have a number common fields. The standard fields are:
Field | Description |
---|---|
|
The Internal DB UUID for this object. This is used for internal references between objects in SimpleMES. (database primary key) |
|
One or more primary key fields for the object. These are usually unique and
provided by the user. The user will typically retrieve the records by these
key fields. The first key field is usually named the same as the object
(e.g. Order has a key field of |
|
The displayed title of the object. This is typically a short one line description of the object. This is displayed in most cases in parentheses along with the code field. |
|
The internal version of this object. This allows optimistic locking of the object. This is incremented on each save and prevents one user from overwriting another user’s data. |
|
The date/time this object was created (not all objects). |
|
The date/time this object was last updated (not all objects). |
Dashboards
General Scan Dashboard Optional Methods
Activities Complete Activity Start Activity Reverse Complete Activity Reverse Start Activity Work Center Selection Work List Activity
A dashboard is an instrument panel that gives your users information and allows them to control some parts of your application. Typically, this dashboard allows configuration so that your users can tailor the display and behavior to their needs. These dashboards can display one or more pages in a resizable set of panels so that a lot of information can be visible to your users to operate on.
Scan Dashboard Optional Methods
Some special-purpose dashboards use optional methods in some activities to coordinate
between those activities and the server. For example, the
Scan Dashboard
will work with the main scan dashboard display.ftl
activity to pass the 'current' order/LSN to the server for processing of the scan.
This is done through the providedScanParameters()
mechanism.
Provided Scan Parameters
The Scan Dashboard uses a main display page to hold the current state of the GUI. The current order/LSN is passed to the server along with the scanned barcode for processing. This is done by implementing an optional method in the display.ftl page that returns the current order and/or LSN. These will then be added to the ScanRequest as sent to the server.
You will probably not need to implement this method, but it is available for other modules to use if needed. In the display.ftl, this is how the method is implemented.
An example:
1
2
3
4
5
6
7
<script>
${params._variable}.provideScanParameters = function(event) { (1)
return {
order: $('#order').val(), (2)
}
}
</script>
1 | The provideScanParameters() method (unique to each panel, so the params._variable
variable is used to make the name unique. This also makes it available in the
global javascript name space for the dashboard to access. |
2 | Grabs the current value of the input field an returns it in a javascript object. |
Dashboard Activities
Complete Activity
uri: /work/completeActivity
This Complete activity is a
Non-GUI Activity .
This means it normally does not display a GUI and just performs the complete action.
This activity works with the
WorkController
complete()
method to perform the action.
The complete activity uses the Order/LSN parameter from the Work Center Selection
activity and attempts to complete the work on it. The Order/LSN is passed to the
complete()
method in the barcode
field in the
CompleteRequest .
Any errors are displayed in the dashboard’s message area.
GUI Events
This activity will trigger these GUI events:
-
ORDER_LSN_STATUS_CHANGED - Sent when the order/LSN is complete.
Provided Parameters Used
This activity uses the parameters provided by other activities:
-
order - The order/LSN from the input field (typically from the Work Center Selection activity).
Start Activity
uri: /work/startActivity
This Start activity is a
Non-GUI Activity .
This means it normally does not display a GUI and just performs the start action.
This activity works with the
WorkController
start()
method to perform the action.
The start activity uses the Order/LSN parameter from the Work Center Selection
activity and attempts to start the work on it. The Order/LSN is passed to the
start()
method in the barcode
field in the
StartRequest .
Any errors are displayed in the dashboard’s message area.
GUI Events
This activity will trigger these GUI events:
-
ORDER_LSN_STATUS_CHANGED - Sent when the order/LSN is started.
Provided Parameters Used
This activity uses the parameters provided by other activities:
-
order - The order/LSN from the input field (typically from the Work Center Selection activity).
Reverse Complete Activity
uri: /work/reverseCompleteActivity
This Reverse Complete activity is a
Non-GUI Activity .
This means it normally does not display a GUI and just performs the complete action.
This activity works with the
WorkController
reverseComplete()
method to perform the action.
The reverse complete activity can use the Order/LSN parameter from the Work Center Selection
activity and attempts to reverse a previous complete on it. The Order/LSN is passed to the
reverseComplete()
method in the barcode
field in the
CompleteRequest .
Any errors are displayed in the dashboard’s message area.
This reverse action will mark the Order/LSN as not done and issue a negative ProductionLog record. This action also places the Qty back in queue. It does not perform a start on the qty.
This activity is also used as an Undo action for the Start Activity.
GUI Events
This activity will trigger these GUI events:
-
ORDER_LSN_STATUS_CHANGED - Sent when the order/LSN complete is reversed.
Provided Parameters Used
This activity uses the parameters provided by other activities:
-
order - The order/LSN from the input field (typically from the Work Center Selection activity).
Reverse Start Activity
uri: /work/reverseStartActivity
This Reverse Start activity is a
Non-GUI Activity .
This means it normally does not display a GUI and just performs the start action.
This activity works with the
WorkController
reverseStart()
method to perform the action.
The reverse start activity can use the Order/LSN parameter from the Work Center Selection
activity and attempts to reverse a previous start on it. The Order/LSN is passed to the
reverseStart()
method in the barcode
field in the
StartRequest .
Any errors are displayed in the dashboard’s message area.
This activity is also used as an Undo action for the Start Activity.
GUI Events
This activity will trigger these GUI events:
-
ORDER_LSN_STATUS_CHANGED - Sent when the order/LSN start is reversed.
Provided Parameters Used
This activity uses the parameters provided by other activities:
-
order - The order/LSN from the input field (typically from the Work Center Selection activity).
Work Center Selection
uri: /selection/workCenterSelection
This activity is designed to be used in the primary section (top) of the dashboard and will allow the operators to select the work center they are working in and enter an order or LSN to process. The buttons that can be added to this activity will let the operator perform common actions on the entered work center, order or LSN.

In this dashboard activity, the operator can change the work center by clicking on the work center. This will open a dialog that lets the operator select the work center they are working in. This work center is retained and used as the default the next time the user uses this dashboard. The work center can also be passed in on the URI for the dashboard. This URI value will supersede the user preference value.
Supported |
Feature |
Configurable Dashboard Buttons |
|
User Configurable (Designer Role) |
GUI Events
This activity will trigger these GUI events:
-
ORDER_LSN_CHANGED - Sent when the user changes the order/LSN field value manually.
-
WORK_CENTER_CHANGED - Sent when the work center is changed.
This activity will handle these events:
-
ORDER_LSN_CHANGED - Sent when the user selects an entry in the work list.
Input Parameters
The default work center can be passed in on the main dashboard URI:
uri: /dashboard?category=OPERATOR&workCenter=WC27
This will open the default dashboard defined for the OPERATOR category with the work center WC27 entered.
Parameters Provided
This activity will provide these parameters to other activities:
-
order
- The input value from the Order/LSN field. -
workCenter
- The current work center.
Javascript Details
This activity creates a few fields used by the javascript logic for this activity:
Field |
Description |
order |
Main input text field that holds the order/LSN input value. |
workCenter |
Hidden field that contains the current work center. |
workCenterDisplay |
A span that holds the current work center for display in the page. |
Work List Activity
uri: /workList/workListActivity
This Work List activity is used to display active or queued work for the operator. The current Work Center (Work Center Selection) is used to filter this work to manageable level, but it is not required.

As the operator selects entries, the current selection is coordinated with the selection activity to keep the actions in synch.
Supported |
Feature |
Configurable Columns |
|
User Configurable (Designer Role) |
GUI Events
This activity will trigger these GUI events:
-
WORK_LIST_SELECTED - Triggered when the user changes the selection in the work list.
This activity will handle these events:
-
ORDER_LSN_CHANGED - Sent when the user changes the order/LSN field value manually. This updates the selected entry(s) in this list.
-
WORK_CENTER_CHANGED - Sent when the user changes the Work Center field value manually. This updates the list elements.
Parameters Provided
This activity will provide these parameters to other activities:
-
selectedOrdersLSNs
- The select orders/LSNs.
Dashboard Events
This section will describe the dashboard events provided for the MES dashboards. These events allow the activities to work together to provide a simple looking GUI that reduces the effort to get things done. See framework Dashboard API for an overview of events and example event handlers.
ORDER_LSN_CHANGED
This event is triggered when the user changes the Order/LSN in the Work Center Selection activity. This event can be used to synchronize all activities with the currently selected order/LSNs.
This is typically used by many activities to update their display for the new selection.
Event Properties
The main properties of the event are:
-
type
- 'ORDER_LSN_CHANGED'. -
source
- The source of the event. Usually the name of the dashboard activity (e.g. 'workCenterSelection'). -
list
- The list of selected orders and/or LSNs.-
order
- The selected order from the list. (String) -
orderID
- The selected order’s ID from the list. (Number) -
lsn
- The selected LSN. Might be undefined. (String) -
lsnID
- The selected LSN’s ID. Might be undefined. (Number) -
operationSequence
- The selected row’s operation sequence. Only populated from WORK_LIST_SELECTED. (Number) -
product
- The product the Order/LSN represents. Only populated from WORK_LIST_SELECTED. (String) -
productID
- The product’s ID. Only populated from WORK_LIST_SELECTED. (Number) -
qtyInQueue
- The quantity in queue. Only populated from WORK_LIST_SELECTED. (Number) -
qtyInWork
- The quantity in work. Only populated from WORK_LIST_SELECTED. (Number)
-
This event can be triggered by user input to the field or when the WORK_LIST_SELECTED is received. Not all fields are populated when triggered by the user input.
ORDER_LSN_STATUS_CHANGED
This event is triggered when the status of an Order/LSN is changed. This event can be used to update the status displayed by other activities.
Event Properties
The main properties of the event are:
-
type
- 'ORDER_LSN_STATUS_CHANGED'. -
source
- The source of the event. Usually the name of the dashboard activity (e.g. 'startActivity'). -
list
- The list of orders and/or LSNs that changed their status.-
order
- The order with the status change. (String) -
lsn
- The LSN with the status change. (String: Optional)
-
Event Sources
This event can be triggered by these activities:
WORK_CENTER_CHANGED
This event is triggered when the work center Work Center Selection activity is changed. This event is be used to synchronize all activities with the currently selected order/LSNs.
This is typically used by many activities to update their display for the new selection.
Event Properties
The main properties of the event are:
-
type
- 'WORK_CENTER_CHANGED'. -
source
- The source of the event. Usually the name of the dashboard activity (e.g. 'workCenterSelection'). -
workCenter
- The new work center (String).
WORK_LIST_SELECTED
This event is triggered when the user changes the work selection changes in the Work List Activity activity. This event can be used to synchronize all activities with the currently selected order/LSNs.
This is typically used by the Work Center Selection activity to populate the Order/LSN field.
Event Properties
The main properties of the event are:
-
type
- 'WORK_LIST_SELECTED'. -
source
- The source of the event. Usually the name of the dashboard activity (e.g. 'workList'). -
list
- The list of selected elements.-
order
- The selected order from the list. (String) -
orderID
- The selected order’s ID from the list. (Number) -
lsn
- The selected LSN. Might be undefined. (String) -
lsnID
- The selected LSN’s ID. Might be undefined. (Number) -
operationSequence
- The selected row’s operation sequence. Might be undefined. (Number) -
product
- The product the Order/LSN represents. Might be undefined. (String) -
productID
- The product’s ID. Might be undefined. (Number) -
qtyInQueue
- The quantity in queue. (Number) -
qtyInWork
- The quantity in work. (Number)
-
Scan Actions
The actions triggered by the scan processing in the ScanService . A scan action is used to tell the client to execute a specific client-side action due to some processing on the server. This includes actions such as pressing a button in the GUI, refreshing due to status changes and to indicate what order/LSN was scanned.
These actions are generally published as a dashboard event so all activities can receive these actions. In general, the scan dashboard will handle these action requests. See Dashboard Events for an overview.
These actions may be related to similarly name dashboard events. For example, the OrderLSNChangeAction will likely trigger the ORDER_LSN_CHANGED event after the change has happened on the dashboard. |
ButtonPressAction
The ButtonPressAction action tells the client dashboard to press a configured dashboard button. This is generally triggered by a scan of an encoded button ID. The internal format is:
^BTN^START
This barcode prefix (BTN) is used to tell the client to press a button. This is used to avoid keyboard/mouse input in a scan-oriented dashboard. See Barcode Formats for more details on general formats.
Scan Action Properties
The main properties of the scan action are:
-
type
- BUTTON_PRESS -
button
- The button ID.
There is a special button ID _UNDO that will trigger the undo action. This is not a true dashboard configured button, but it works the same. |
OrderLSNChangeAction
The OrderLSNChangeAction is used to notify the client that the current order/LSN needs to be changed in the client. This is used when an order/LSN is scanned should be the 'current' order to be processed in the client.
This server-side action is used to trigger the client-side dashboard event: ORDER_LSN_CHANGED. The event details are listed there.
OrderLSNStatusChangedAction
The OrderLSNStatusChangedAction is used to notify the client that the status of an order/lsn has changed. Typically, this is used by specific dashboard activities to refresh the displayed status of the current order.
This server-side action is used to trigger the client-side dashboard event: ORDER_LSN_STATUS_CHANGED. The event details are listed there.
API
The Groovy API for the SimpleMES contains details on all fields and methods for most objects/services.
The API in SimpleMES is based on Groovy/GORM Domain objects and Services. These are accessed like normal Java/Groovy classes. Refer to the GORM documentation for general access to the Domain objects and Services.
The mechanics of accessing the API via HTTP is covered in more detail in the Enterprise Framework.
Stable API
Key services in SimpleMES are considered 'Stable APIs' . This means the SimpleMES developers will make every effort to retain backward compatibility with these services. This does not mean that there will be no changes to these APIs. It means that most of the changes will not affect older clients who use these APIs. Typically, later releases will add new, optional fields to the requests. The returned objects may have new fields added or some existing fields may become optional. There is always a chance that a breaking change will be needed, but this will rarely happen.
These Stable APIs are flagged in the Doc pages with the note: Stable API .
Your code should try to use these Stable APIs and the domain objects whenever possible. This will improve the life of your code. |
REST
These services are frequently exposed via HTTP with a REST (Representational state transfer) approach. All actions are performed using HTTP requests with specific URLs and content.
Order/LNS GET APIs
A common type of API is the normal REST GET method. This is used get information about various objects in
the system. It is used frequently to get data on Orders or LSNs. When used in places like the OrderController,
the URL can be used with an LSN, order. It can be the ID (numeric) or the order/LSN name (primary key value).
For example, the determineQtyStates()
method can be called for an order using the name:
http: GET
url: /order/determineQtyStates/M1001
{
"id":1000000001308000,
"product":"PRODUCT_XYZ",
"title":"Standard 27\" Bicycle"
}
This type of method uses a standard resolution precedence:
-
LSN - ID (numeric)
-
Order - ID (numeric)
-
LSN - Name
-
Order - Name
CRUD
In general, Create, Read, Update, Delete (CRUD) actions are handled by REST-style URLs and actions on the object’s controller page. For example, to read a Product, the client will perform an HTTP GET on the URL:
/product/crud/BIKE27
This will return the JSON formatted data for the product BIKE27:
{
"id":1000000001308000,
"product":"PRODUCT_XYZ",
"title":"Standard 27\" Bicycle"
}
Services
Most Non-CRUD actions are accessed via the Services in SimpleMES. These services are normal Micronaut-style singleton services and provide most of the complex features in SimpleMES. The built-in GUIs in SimpleMES always access the the business functions via these Services or the Controller/Domain classes.
These Services always have a corresponding controller to allow HTTP access to these Services. For example, the
WorkService.start()
method has a controller at the URI /work/api/start
. This controller URI expects JSON content
that matches the method’s input parameter (StartRequest
in this case).
These requests follow the general REST approach, but primarily use the HTTP POST verb. These APIs also do not use the HTTP URL to identify the objects being processed in most cases. The HTTP request content defaults to JSON format and it will contain the objects to be processed. The URL does not reference the object being affected since multiple objects may be involved.
These types of API calls are always implemented as a normal Micronaut Service. The details of the Service are fully documented in the Groovy API docs for the Service itself. There is a controller associated with the service and provides the REST-style HTTP access. Internal programs running within the SimpleMES application server should use the service directly. The HTTP API is only intended for access from external clients (GUIs, machines, etc).
These services always end with 'Service'. For example, WorkService
, OrderService
, etc.
An example of this service is the WorkService start()
method.
To perform a start action on an Order, the following request is used:
http: POST
url: /work/start
{
"order":"100023",
"qty":10.0
}
Domains
Demand LSN LSNSequence Order OrderSequence
Floor WorkCenter
Numbering CodeSequence
Tracking ActionLog ProductionLog
LSN
An LSN (Lot/Serial Number) is a lightweight element of an Order. This is the finest level of tracking possible in SimpleMES. An LSN has a quantity and a status. A quantity of 1.0 means this is a a serial number. The default quantity for an LSN is defined at the Product level.
See LSN for an overview.
Tracking within SimpleMES is performed mainly at the order level, but it is possible to track at finer levels as needed. You may track work at these levels, interchangeably:
-
Order Only
-
Order and LSN
-
LSN Only
LSNs may be assigned at order release time or later as needed. LSNs may be globally unique or can be unique within a given Product. SimpleMES will attempt to find the appropriate LSN and some GUIs will prompt the user if multiples are found. In most cases, you may also use an optional quantity when processing work on the shop floor.
The WorkService is used to determine what levels an order/LSN can be processed
at. The basic start()
and complete()
actions are defined within this service.
LSNs
can’t be imported on their own. They can be imported, exported, changed and deleted as
part of the Order
. You will use the normal SimpleMES API for this.
Fields
The fields defined for this object include:
Field | Description |
---|---|
order |
The order the LSN belongs to.(key field) |
lsn |
The lot/serial number’s name. During creation, if the |
status |
The LSN’s overall status. Other fields determine the finer-grained status at various stages or processing. See Status for an overview. |
qty |
The basic build quantity for the LSN. This is the number of pieces to be built. |
qtyInQueue |
The quantity in queue waiting to be processed. |
qtyInWork |
The quantity currently in work (being processed). |
qtyDone |
The quantity that has been completed for this LSN. |
Because this LSN has a combination primary key of order + lsn , the API calls using
JSON need
to be a little more complex. For example:
|
{
"order":"M1001",
"lsn":"SN2001"
}
If the lsn
(SN2001 above) is unique, then you can leave off the order
element.
LSNSequence
LSN sequences control how LSNs keys are automatically generated for an Order. The format of these LSNs is quite flexible. The LSN can be based on the order, the date or other related values.
The format string can contain replaceable parameters that are used to generate a unique or semi-unique LSN. These replaceable parameters are listed as $order in the format string. At generation time, these strings are replaced by the actual value in use at the time.
The replaceable parameters for LSNs are:
Parameter | Description |
---|---|
$currentSequence |
A semi-unique sequence that is incremented after each code is generated. |
$order.order |
The parent order for this LSN. |
$product.product |
The product that the parent order is to build. |
Standard Parameters |
All of the standard parameters for a CodeSequence (e.g. |
These order and product parameters are the full order and product objects. This means you can use fields of those objects in creation of the LSN strings. For example:
${order.product?.product ?: 'DEFAULT'}
This will use the product in the format string. If the order has no product, then the string 'DEFAULT' will be used.
The question mark ('?') syntax is used to avoid null pointer exceptions in the code. The '?:' syntax is standard Groovy syntax which means: if the left side is null, then use the right side.
Order
An Order is a manufacturing order used to produce an (optional) Product. These orders specify the quantity needed and optionally a due date, start date and other information. Orders may use the standard Routing or may have an order-specific Routing.
Orders can be imported, exported, changed and deleted using the standard SimpleMES REST API.
See Order for an overview.
Fields
The fields defined for this object include:
Field | Description |
---|---|
order |
The order’s name. During creation, if the |
overallStatus |
The order’s overall status. Other fields determine the finer-grained status at various stages or processing. See Status for an overview. |
product |
The Product to be built by this order. |
qtyToBuild |
The number of pieces to be built for this order. Default 1.0. |
qtyInQueue |
The number of pieces in queue to be worked. This is only used if there is no routing for the order. |
qtyInWork |
The number of pieces actively in work. This is only used if there is no routing for the order. |
qtyDone |
The number of pieces already done (finished) for this order. |
lsns |
The LSN’s that are part of this order. Not all orders will track LSN’s. This is configurable at the Product level. |
dateCompleted |
The date this order was completed (marked as Done). |
OrderSequence
Order sequences control how Orders keys are automatically generated for an Order that has no order value. The format of these Orders is quite flexible. The LSN can be based on the product, the date or other related values.
The format string can contain replaceable parameters that are used to generate a unique or semi-unique Order key. These replaceable parameters are specified like $product.product in the format string for the sequence definition. At generation time, these strings are replaced by the actual value in use at the time.
The replaceable parameters for Orders are:
Parameter | Description |
---|---|
$currentSequence |
A semi-unique sequence that is incremented after each code is generated. |
$product.product |
The product that the parent order is to build. |
Standard Parameters |
All of the standard parameters for a CodeSequence (e.g. |
${product?.product ?: 'ACME'}
This will use the product in the format string. If the order has no product, then the string 'ACME' will be used.
The question mark ('?') syntax is used to avoid null pointer exceptions in the code. The '?:' syntax is standard Groovy syntax which means: if the left side is null, then use the right side.
WorkCenter
A WorkCenter is a machine or location where a manufacturing operation is performed. It can also refer to the people at a location where the operation is performed.
Work centers can also be used to partition operations on a Routing to limit where some operations can be performed.
See WorkCenter for an overview.
Fields
The fields defined for this object include:
Field | Description |
---|---|
workCenter |
The work center name (key field). |
title |
The title (short description) of the work center. |
overallStatus |
The overall status of the work center. This is a BasicStatus. See Status for an overview. |
CodeSequence
CodeSequenceTrait control how various system generated key values are generated within SimpleMES. There are a number of specific classes used to generate keys for objects such as LSN’s or Orders. This section covers features common to all code sequences. Specific sequences such as LSNSequence inherit these features.
The sequence is defined using a format string and an optional sequence number. The format string can be a simple value or it can contain replaceable parameters that are replaced at generation time. For example, to generate some codes/keys for the form XYZ-123, you would use the format string:
XYZ-$currentSequence
This will result in codes like 'XYZ-001','XYZ-002'…
At generation time, these '$' strings are replaced by the actual value in use at the time. These '$' strings follow the standard Groovy G-String formats. There are a number of standard parameters that can be used in all code sequences:
Parameter | Description |
---|---|
$currentSequence |
Standard sequence counter. This is incremented after each code is generated. |
${date.format('yyMMdd')} |
The date/time the code was generated. The various format strings are shown below. |
This list is much larger for the specific sequences such as LSNSequence.
The simple format '$pogo.field' is not supported. You should use the format with curly brackets version '${pogo.field}' |
Date Formats
The common date/time formatting strings are shown below. The number of characters
String | Description | Example |
---|---|---|
y |
Year |
1996; 96 |
M |
Month in year |
July; Jul; 07 |
w |
Week in year |
27 |
W |
Week in month |
2 |
D |
Day in year |
189 |
d |
Day in month |
10 |
F |
Day of week in month |
2 |
E |
Day in week |
Tuesday; Tue |
a |
Am/pm marker |
PM |
H |
Hour in day (0-23) |
0 |
k |
Hour in day (1-24) |
24 |
K |
Hour in am/pm (0-11) |
0 |
h |
Hour in am/pm (1-12) |
12 |
m |
Minute in hour |
30 |
s |
Second in minute |
55 |
You can use as many digit characters as needed. Some format strings use abbreviation for shorter values. For example, MMM will be displayed as Jul. See the Java class SimpleDateFormat for full details.
More complex logic can be used in these format strings if needed. For example, this format:
${def d=date+1;d.format('yyMMdd')}
This will format tomorrow’s date into the yyMMdd format. The length of the format string is limited, so complex script logic should be avoided.
Product
A Product is a part or object that is produced on your shop floor or purchased from external sources. This is sometimes known as a part number or model number. This product defines the optional Routing needed to produce the product.
Products can be imported, exported, changed and deleted using the standard SimpleMES REST API.
See Product for an overview.
Fields
Field | Description |
---|---|
product |
The Product name.(key field) |
title |
The title (short description) of the product. |
lsnTrackingOption |
Defines if LSNs are used with the product. Affects how orders can use LSNs. |
lsnSequence |
The LSN sequence to use. If not specified, then LSNs will not be created for the order on creation. If not defined, then no LSNs will be automatically created for orders. |
lotSize |
The lot size (size of child LSN if LSNs are used). |
operations |
The list of operations, if this Product needs a Product-level routing. |
masterRouting |
Defines a shared master routing to be used to produce this product.
This is ignored if the Product-level |
Routing
All routings in SimpleMES have some common characteristics. They have a list of operations and support some common methods See RoutingTrait for details.
These operations can be simple actions such as ASSEMBLE or TEST. They may also be a composite operation that is made up of several actions that are not tracked individually within SimpleMES.
Some examples operations are:
-
10 - Assemble
-
20 - Paint
-
30 - Pack
-
40 - Test
The routing may be a master routing that can be used on multiple products or it may be specific to a single product. This decision is made when defining the product.
Routings can be imported, exported, changed and deleted using the standard SimpleMES REST API. The specific routing for a product can be imported as part of the product import.
Master Routing
The MasterRouting is a pre-defined routing that is used for more than one Product. These
have a routing
key field and a title
field. These master routings are maintained in the
Master Routing definition GUIs.
Product Routing
The Product has a list of operations and implements the RoutingTrait. It is used for the single product.
Order Routing
The Order has a copy of the list of operations from the Master or product routings.
Fields
The most important fields include:
Field | Description |
---|---|
routing |
The routing name (key field, MasterRouting Only). |
title |
The title (short description) of the routing (MasterRouting Only). |
operations |
The list of sorted operations for this routing. See OperationTrait below. |
OperationTrait
A OperationTrait is a single operation needed to manufacture a Product. These operations can be simple actions such as ASSEMBLE or TEST. They may also be a composite operation that is made up of several actions that are not tracked within SimpleMES.
The primary key for the operation is the sequence
(an integer). The title
is used
mainly for display purposes.
Field | Description |
---|---|
sequence |
The sequence the operation should be performed in (key field, integer). |
title |
The title (short description) of this operation. This is usually visible to the production operator. |
ActionLog
The ActionLog class is used to record all important actions for a specific element (e.g. Order or LSN). Actions such as Release, Start and Complete a logged here.
These records are designed to exist as long as the owning objects (e.g. Order) are in the database.
The records are archived when the owning objects are archived. This is something the Order object
handles with its findRelatedRecords()
method to find the ActionLog records.
See ActionLog for an overview.
ProductionLog
The ProductionLog class represents the result of a production action on the shop floor. Typically, this is written when the order/LSN is taken out of work so that the elapsed time can be calculated. This includes actions like complete and reverse start.
These records are designed to exist without direct references to other domain objects. This means the references use the primary key field for the object (e.g. Order, LSN, etc). Those referenced objects can be archived and these production log records can be kept in the database longer than the other domain objects if needed.
See ProductionLog for an overview and details on archiving.
Services
Demand Services OrderService WorkListService WorkService
System Services ScanService
Tracking Services ProductionLogService
Introduction
SimpleMES provides much of the business logic using 'services'. These services use the domain objects to affect the persistent state of objects. Most of the MES business logic is handled in the services.
Demand Services
This demand package provides objects to handle demand for production within the shop floor. This includes handle work on the shop floor and dealing with orders from customers.
OrderService
The OrderService is used to perform key actions on the entire Order. This includes releasing the order and other global tasks.
This service’s methods are exposed by the OrderController . This controller supports the request/response POGOs in JSON format.
release()
The release()
method is used to release an order to the shop floor for processing. The entire order or
a portion of the order can be released. Automatic LSN generation, if needed, occurs at release time.
See LSN for details on when LSNs are automatically generated.
This method is part of the Stable API . |
OrderReleaseRequest
This is used to specify the order and optional quantity to release.
The fields defined for the OrderReleaseRequest include:
Field | Description |
---|---|
order |
The order to release (Required). |
qty |
The quantity to release. (Default: all remaining quantity). |
OrderReleaseResponse
This is used to return the order (in released state) to the caller.
The fields defined for this OrderReleaseResponse include:
Field | Description |
---|---|
order |
The order released. |
qty |
The quantity released. |
Order Service Extension Points
Many other modules may need to extend core methods with module-specific logic. We allow this using the framework’s Extension Points feature. The extension points in this service include:
-
OrderService.release() - The Order release() method. OrderReleasePoint
All of the extension points in this module are listed at Service Extension Points .
WorkListService
The WorkListService is used to find work that is available (in queue) or in work. This list can be restricted to work in a work center or global. This can show Orders and/or LSNs.
This service’s methods are exposed by the WorkListController . This controller supports the request/response POGOs in JSON format.
findWork()
This is the primary method in this services. It finds work that is in queue or in work with various filter criteria. This method returns a list of Orders/LSNs that match the criteria. The list is row-limited with the normal offset and max settings. This response also includes the total number of rows available, for support of paging.
This method performs 4 queries to find all types of work needed:
-
Orders with no routing.
-
Orders with a routing.
-
LSNs with no routing.
-
LSNs with a routing.
Most customers will use one of these combinations, but the MES will not limit you to just one. This means all four queries will be executed and combined.
This method is part of the Stable API . |
FindWorkRequest
The fields defined for the FindWorkRequest include:
Field | Description |
---|---|
workCenter |
The work center to filter the results by. Only work in this work center will be displayed (Optional). |
findInWork |
If true, then the |
findInQueue |
If true, then the |
max |
The maximum number of rows to return (Default: 10). See Max Rows and Offset. |
offset |
The row to start the results sets from (Default: 0). |
FindWorkResponse
The fields defined for this FindWorkResponse include:
Field | Description |
---|---|
totalAvailable |
The total number of rows available that matches the search criteria. (int). |
list |
The list of details rows found that match the search criteria. This detail represents a single unit in queue or in work. This can be an order and/or LSN. (List of FindWorkResponseDetail). |
FindWorkResponseDetail
The fields defined for this FindWorkResponseDetail include:
Field | Description |
---|---|
order |
The order that is in work or queue (Order). |
lsn |
The LSN that is in work or queue (LSN). |
operationSequence |
The operation this qty is at (0 means not at an operation) (int). |
qtyInQueue |
The qty in queue (BigDecimal). |
qtyInWork |
The qty in queue work (BigDecimal). |
workCenter |
The work center the unit is in (WorkCenter). |
inWork |
True if the unit is in work (boolean). |
inQueue |
True if the unit is in queue (boolean). |
Max Rows and Offset
Each of the 4 queries can return the max number of rows specified in the request (e.g. 10 rows each). The service will combine these results into one list that may be up to 4 times larger than the max rows setting. This is rare since most customer don’t use all 4 types of tracking levels (Orders/LSN/routing).
The offset will be used to support paging.
The max value has an absolute maximum as defined in Row Limits (typically 100).
WorkService
The WorkService is used start/complete that is available.
This service’s methods are exposed by the WorkController . This controller supports the request/response POGOs in JSON format.
start()
The start() method is used to begin work on an order or LSN. This can be the entire order, one LSN or just a portion. The work is performed at an optional work center and is logged to the action log.
The StartRequest object is the main argument for the
start()
. This is a simple POGO that you must populate before you perform the start.
This method returns a list of the StartResponse objects with the results of the start action.
This method is part of the Stable API . |
The basic flow for this start()
method uses these key objects and methods. These methods can be
important extension points (see Enterprise Framework
for details).
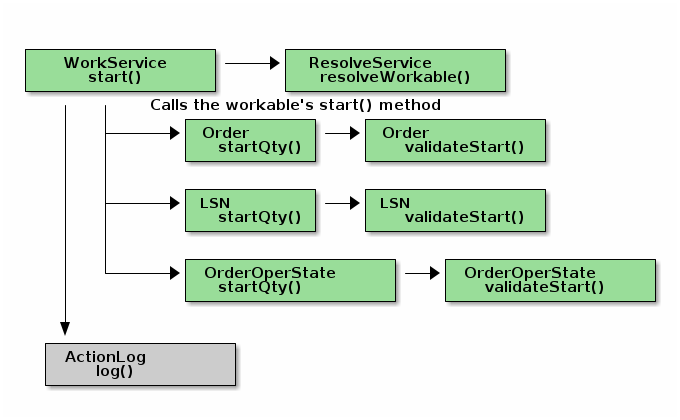
The start()
finds the appropriate
Workable
that matches the start request’s inputs (e.g. an Order or sub-element that can be started). The Work service then
calls the startQty()
on that Workable. This then validates that the Qty can be started.
If everything passes the validation, then the WorkService will log the start via the ActionLog.
There is a corresponding reverseStart()
method to "un-start" the work.
See WorkStateTrait for common qty/date manipulation logic that applies to all supported complete scenarios (e.g. order, LSN and routing variations).
StartRequest
The fields defined for the StartRequest include:
Field | Description |
---|---|
barcode |
A generic barcode or user input that can be an order or LSN. |
order |
The Order to be processed. |
lsn |
The LSN to be processed. |
qty |
The number of pieces to be processed. |
operationSequence |
The sequence of the operation (step) the order/LSN is to be processed at. |
workCenter |
The WorkCenter this work is being performed at. |
StartResponse
The fields defined for the StartResponse include:
Field | Description |
---|---|
order |
The Order processed. |
lsn |
The LSN processed. |
qty |
The number of pieces to be processed. |
operationSequence |
The sequence of the operation (step) the order/LSN was processed at. |
undoActions |
The list of actions needed to undo the start. |
complete()
The complete() is used to finish work on an order or LSN. This can be the entire order, one LSN or just a portion. The work is performed at an optional work center and is logged to the action log and ProductionLog.
The CompleteRequest object is the main argument for the
complete()
. This is a simple POGO that you must populate before you perform the complete.
This method returns a list of CompleteResponse objects with the results of the complete action.
This method is part of the Stable API . |
The basic flow for this complete()
method uses these key objects and methods. These methods can be
important extension points (see Enterprise Framework
for details).
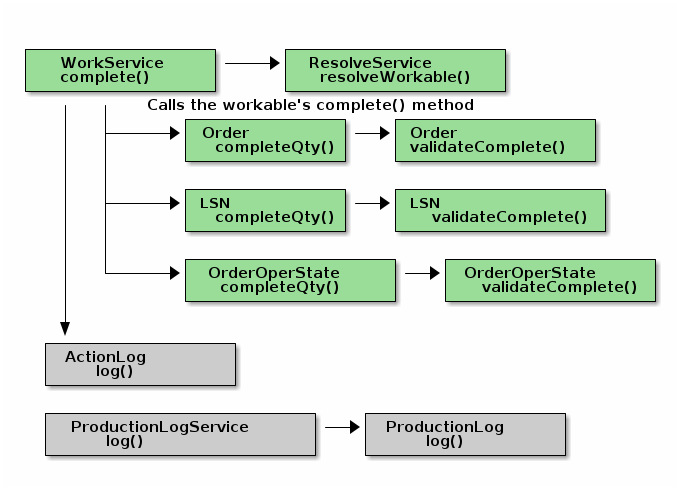
The complete()
finds the appropriate
Workable
that matches the request’s inputs (e.g. an Order or sub-element that can be completed). The Work service then
calls the completeQty()
on that Workable. This then validates that the Qty can be completed.
If everything passes the validation, then the WorkService will log the complete via the ActionLog and ProductionLog.
See WorkStateTrait for common qty/date manipulation logic that applies to all supported complete scenarios (e.g. order, LSN and routing variations).
CompleteRequest
The CompleteRequest
object is the main argument for the
complete()
. This is a simple POGO that you must populate before you perform the complete.
Field | Description |
---|---|
barcode |
A generic barcode or user input that can be an order or LSN. |
order |
The Order to be processed. |
lsn |
The LSN to be processed. |
qty |
The number of pieces to be processed. |
operationSequence |
The sequence of the operation (step) the order/LSN is to be processed at. |
workCenter |
The WorkCenter this work is being performed at. |
CompleteResponse
The fields defined for the CompleteResponse include:
Field | Description |
---|---|
order |
The Order processed. |
lsn |
The LSN processed. |
qty |
The number of pieces to be processed. |
operationSequence |
The sequence of the operation (step) the order/LSN was processed at. |
done |
If true, then the completed unit is done (no more processing planned). |
undoActions |
The list of actions needed to undo the complete. |
ScanService
The ScanService is used process scanned input from the client, usually from a barcode scanner. This includes releasing an order to the shop floor.
This service’s methods are exposed by the ScanController . This controller supports the request/response POGOs in JSON format.
scan()
The scan()
method handles the scan from the user. This resolves the ID and will sometimes process the ID.
This core module will attempt to start and order/LSN if it is in queue.
This method is part of the Stable API . |
ScanRequestInterface
This is used to specify the barcode text to be processed.
The fields defined for the ScanRequestInterface include:
Field | Description |
---|---|
barcode |
The barcode input to process (Required). |
ScanResponseInterface
This is used to return what was processed for the barcode input. This includes suggested client actions and any messages from the actions taken by the scan service on the server (e.g. Start, etc).
The fields defined for the ScanResponseInterface include:
Field | Description |
---|---|
barcode |
The barcode input processed. |
resolved |
True if the barcode matched a valid object and the object was processed (Boolean) |
order |
The order the scan resolved to. |
lsn |
The LSN the scan resolved to. |
operationSequence |
The operation sequence processed (if an operation was used). |
scanActions |
The recommended actions the client will need to take to respond to the scan. This can include button presses or Dashboard event triggering (List<Map>). See ScanActionInterface |
messageHolder |
Messages from the actions performed by the scan service logic (e.g. from the Start, Complete, etc). |
Example
JSON is supported with the normal HTTP POST request for the scan, using this URI:
/scan/scan
The request content in JSON format is shown below:
{
"scanRequest":{
"barcode":"M1001"
}
}
The response in JSON format is shown below:
{
"scanResponse": {
"barcode": "M1001",
"operationSequence": 0,
"resolved": true,
"order": { (1)
"class": "org.simplemes.mes.demand.Order",
"id": 32,
"order": "M1001"
},
"messageHolder": { (2)
"level": "info",
"text": "Started quantity [1.0000] for [M1001]."
},
"scanActions": [ (3)
{
"type": "ORDER_LSN_STATUS_CHANGED",
"order": "M1001"
},
{
"type": "ORDER_LSN_CHANGED",
"order": "M1001",
"qtyInQueue": 0.0000,
"qtyInWork": 1.0000
}
]
}
}
1 | The order that matches the requested barcode. |
2 | The messages from any server-side actions that were performed on scanned element (order). |
3 | The suggested client-side actions to perform. This includes an update to the order status and the current order was changed. |
ProductionLogService
The ProductionLogService provides a way to log production actions on the shop floor for use in reporting. This typically helps provide the production rates and yield data for common reports.
This service’s methods are not exposed by any controller. They are designed to be called from other services.
log()
The log()
method will create a single Production Log record for the requested inputs.
Minimal checking and processing on the input will be made by the service.
ProductionLogRequest
This is used as the input to the log()
method.
The fields defined for the
ProductionLogService
include these important fields:
Field | Description |
---|---|
action |
The action performed (Required). |
dateTime |
The date/time the action took place (Default: now). |
startDateTime |
The date/time the action took place (Default: dateTime). |
elapsedTime |
The elapsed time in milliseconds for the action (Default: The difference from startDateTime and dateTime or 0). |
user |
The user who performed this action (User ID) (Default: current request user). |
order |
The Order processed. |
lsn |
The LSN processed. |
product |
The Product for the LSN/Order. (Default: The order’s product). |
masterRouting |
The master routing this production action took place on. |
operationSequence |
The routing operation sequence where this action was performed. |
workCenter |
The Work Center this action took place at. |
qty |
The quantity processed during this action (Default: 0.0). |
qtyStarted |
The quantity started that was removed from work on this action (Default: 0.0). |
qtyCompleted |
The quantity completed by this action (Default: 0.0). |
archiveOld()
The archiveOld()
method will find any 'old' production log records and archive/delete them.
The ageDays
value controls which records are considered old. There is also
a mechanism to use a specific date/time for finding the old records for testing.
ProductionLogArchiveRequest
This is used as the input to the archiveOld()
method.
The fields defined for the
ProductionLogArchiveRequest
include these important fields:
Field | Description |
---|---|
ageDays |
The age (in days) used to determine if a record is an 'old' record and eligible for archiving/deleting. Supports fractions (Required). |
batchSize |
The size of the batch used when archiving these records. This determines the database transaction size and the size of the archive JSON file (if records are not deleted) (Default: 500). |
delete |
If true, then the records are deleted, not archived (Default: false). |
asciidoctor-version |
2.0.10 |
safe-mode-name |
unsafe |
docdir |
/home/runner/work/simplemes-core/simplemes-core/mes-core/src/main/docs/asciidoc |
docfile |
/home/runner/work/simplemes-core/simplemes-core/mes-core/src/main/docs/asciidoc/reference.adoc |
doctype |
book |
imagesdir-build |
images |
imagesdir-src |
images |
imagesdir |
images |